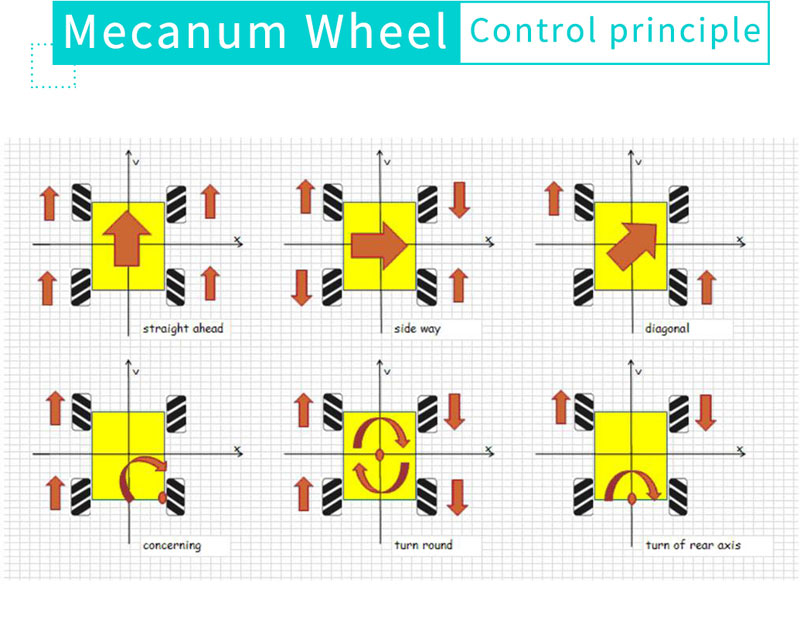
- Open the Onbot Java IDE.
- Create a new project.
- In the project, create a new class called MecanumTeleop.
- In the MecanumTeleop class, add the following imports:
1 2 3 | import com.qualcomm.robotcore.eventloop.opmode.LinearOpMode; import com.qualcomm.robotcore.hardware.DcMotor; import com.qualcomm.robotcore.util.ElapsedTime; |
- In the MecanumTeleop class, declare the following variables:
6 7 8 9 10 | private ElapsedTime runtime = new ElapsedTime(); private DcMotor leftFrontMotor; private DcMotor rightFrontMotor; private DcMotor leftBackMotor; private DcMotor rightBackMotor; |
- In the MecanumTeleop class, override the init() method. In this method, initialize the motors:
11 12 13 14 15 16 17 18 19 20 21 22 | @Override public void init() { leftFrontMotor = hardwareMap.get(DcMotor.class, "left_front_motor"); rightFrontMotor = hardwareMap.get(DcMotor.class, "right_front_motor"); leftBackMotor = hardwareMap.get(DcMotor.class, "left_back_motor"); rightBackMotor = hardwareMap.get(DcMotor.class, "right_back_motor"); leftFrontMotor.setMode(DcMotor.Mode.RUN_WITHOUT_ENCODER); rightFrontMotor.setMode(DcMotor.Mode.RUN_WITHOUT_ENCODER); leftBackMotor.setMode(DcMotor.Mode.RUN_WITHOUT_ENCODER); rightBackMotor.setMode(DcMotor.Mode.RUN_WITHOUT_ENCODER); } |
- In the MecanumTeleop class, override the loop() method. In this method, read the gamepad inputs and drive the robot:
23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 | @Override public void loop() { // Read the gamepad inputs double x = gamepad1.left_stick_x; double y = gamepad1.left_stick_y; double rotation = gamepad1.right_stick_x; // Drive the robot leftFrontMotor.setPower(x + y + rotation); rightFrontMotor.setPower(x - y - rotation); leftBackMotor.setPower(x - y + rotation); rightBackMotor.setPower(x + y - rotation); // Display the runtime telemetry.addData("Runtime", runtime.seconds()); telemetry.update(); } |
- To run the code, click the “Play” button in the Onbot Java IDE.
- The robot should now drive in all directions in response to the gamepad inputs.
Here are some additional tips for creating a mecanum teleop code in Onbot Java:
- Use the ElapsedTime class to keep track of the robot’s runtime. This can be useful for debugging and for timing events.
- Use the telemetry class to display information about the robot’s state on the Driver Station. This can be useful for debugging and for monitoring the robot’s performance.
- Use the Scheduler class to schedule periodic tasks. This can be useful for tasks that need to be run at regular intervals, such as updating the odometry or checking the battery level.
I hope this tutorial has been helpful. If you have any questions, please feel free to ask in the comments.